Explore Fyne, a GUI framework for Go
Programming Snapshot – Fyne
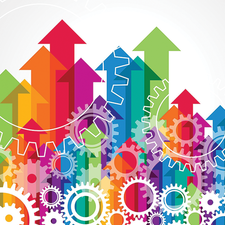
With the Fyne framework, Go offers an easy-to-use graphical interface for all popular platforms. As a sample application, Mike uses an algorithm to draw arrows onto images.
My new favorite language, Go, impresses with its image processing routines, manipulating the pixels in an image just as quickly as ordinary numbers or text [1]. I often paint arrows in digital images, either for illustration purposes or to point out something funny in a snapshot, and I wondered how hard it would be to automate this task. Thus far, I have always had to fire up Gimp, select a path with the Path tool, and then place an arrow in the image with an Arrow plugin that I downloaded from somewhere on the Internet. With Go, there's got to be an easier way!
GUI Preferred
Actually, I prefer command-line tools, but sometimes a traditional graphical interface is just more practical, for example, to select a place in a photo where I want the program to draw a red arrow.
The arrow in Figure 1 illustrates, for example, that the tape measure in the photo, which I found while rummaging through my dusty desk drawers, is an age-old tchotchke from the once thriving company Netscape. Remember them? Yes, the browser manufacturer, where I worked for a few years during the 1990s, shortly after I left Munich and escaped to Silicon Valley "for a year" but somehow never found my way back.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
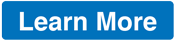
News
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.