Game development with Go and the Fyne framework
Programming Snapshot – Game Development
We all know that the Fyne framework for Go can be used to create GUIs for the desktop, but you can also write games with it. Mike Schilli takes on a classic from the soccer field.
The European soccer championship a year ago was quite a flop for Germany, with what used to be a World Cup-winning squad, but one scene from the Czech Republic's match against Scotland still sticks in my mind. The Scots goalkeeper had run far out of the goal, which Czech player Patrik Schick noticed while hovering at the halfway line. Schick quickly fired the ball into the out-of-bounds goalkeeper's goal with an eye-catching arcing shot. Since then, I've been trying to replicate this feat in my position as striker for the amateur team "Beer Fit" in San Francisco, though without any success so far. This is what prompted me to turn this into a video game written in Go for my Programming Snapshot column.
The underlying physics for the chip shot [1] in soccer is known as "projectile motion," and it's described in any good undergrad physics book. I happen to know this exactly because during my electrical engineering studies I sweated my way through many an exam in the murderous "Technical Mechanics" course. And even many, many years later, holding a totally yellowed degree certificate in my trembling hands, I only needed a short refresher to derive the formulas for the ball position as a function of the starting point, the angle and the velocity of the launch, and the elapsed time.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
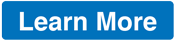
News
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.