Discarding photo fails with Go and Fyne
Programming Snapshot – Go and Fyne
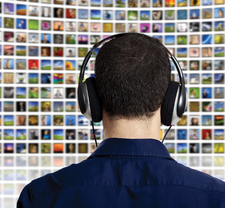
© Lead Image © Erik Reis, 123RF.com
If you want to keep only the good photos from your digital collection, you have to find and delete the fails. Mike Schilli writes a graphical application with Go and the Fyne framework to help you cull your photo library.
Command-line programs in Go are all well and good, but every now and then you need a native desktop app with a GUI, for example, to display the photos you downloaded from your phone and sort out and ditch the fails. At the end of the day, only a few of the hundreds of photos on your phone will be genuinely worth keeping.
Three years ago, I looked at a graphical tool – very similar to the one discussed in this article – that let the user manually weed out bad photos [1]. It ran on the Electron framework to remote control a Chrome browser via Node.js. Recently, the Go GUI framework Fyne has set its sights on competing with Electron and dominating the world of cross-platform GUI development. In this issue, I'll take a look at how easy it is to write a photo fail killer in Go and Fyne.
Recently, ADMIN, a sister publication of Linux Magazine, featured some simple examples [2] with Fyne, but a real application requires some additional polish. Listing 1 [3] shows my first attempt at a photo app that reads a JPEG image from disk and displays it in a window along with a Quit button. The photo dates back to my latest tour of Germany in 2021, where I set out to track down Germany's best pretzel bakers between Bremen and Bad Tölz. Figure 1 shows the app shortly after being called from the command line with a fabulous pretzel from Lenggries on the southernmost edge of Germany. My only complaint about the app's presentation with the photo and the Quit button is that it takes a good two seconds to load a picture from a cellphone camera with a 4032x3024 resolution from the disk and display it in the application window. Building a tool for image sorting with sluggish handling like this wouldn't attract a huge user base.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
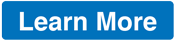
News
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.
-
Linux Kernel 6.15 Now Available
The latest Linux kernel is now available with several new features/improvements and the usual bug fixes.
-
Microsoft Makes Surprising WSL Announcement
In a move that might surprise some users, Microsoft has made Windows Subsystem for Linux open source.
-
Red Hat Releases RHEL 10 Early
Red Hat quietly rolled out the official release of RHEL 10.0 a bit early.
-
openSUSE Joins End of 10
openSUSE has decided to not only join the End of 10 movement but it also will no longer support the Deepin Desktop Environment.
-
New Version of Flatpak Released
Flatpak 1.16.1 is now available as the latest, stable version with various improvements.
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.