Pyro – Networking made simple
Several of my projects have required multiple Raspberry Pis working in tandem to accomplish an ultimate goal, such as driving multiple independent displays or integrating a device with a dedicated controlling computer. Sometimes the setup had unique hardware (e.g., sensors); other times distance made it easier to use a remote system and WiFi rather than a lot of cabling. Although you can choose from many approaches to distributed technology, here, I'll focus on the Python remote objects (Pyro) library.
My most recent project that fell in this category was a set of scoreboards for my church's Vacation Bible School. I integrated four large LCD TVs into the set design (Figure 1) and dedicated a Raspberry Pi to each one. Also, I wanted the ability to update each team's score in real time from a centralized console. To accomplish this, I wrote the code for the scoreboards and their controller in Python and communicated between the different screens with Pyro [1].
Each Pi runs a Raspbian Lite distro and a custom Python script. After flashing the SD card with Raspbian, the only special configuration was to connect to the WiFi network and install the Pyro library. Python is installed by default with Raspbian, and the PyGame library, also a default, provided the graphics. PyGame can drive the Pi framebuffer directly, so the graphical desktop isn't needed.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
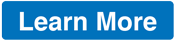
News
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.