Building a hobby OS with Bochs and Qemu
Everyone who works professionally with Linux is used to building software from the source code, perhaps implementing small changes, automating routine work with shell scripts, or developing their own software in one of the many current programming languages. Tinkering with your own, completely new operating system, on the other hand, is a pretty unusual pastime. If you start from scratch, it will take a long time before your system is useful for anything.
If you are looking for a challenging amateur project (for example, if you are a computer science student) or you want a better understanding of the theoretical basics of interrupts, memory management, scheduling, and other OS features, working on your own kernel can provide valuable insights. Linus Torvalds actually created Linux through a similar tinkering project. In 1991, he posted in a Minix news group, "I'm doing a (free) operating system (just a hobby, won't be big and professional like gnu) for 386(486) AT clones." [1]
A development environment for an operating system is more complex than one for an application, because you cannot simply compile the source code and run it on a trial basis. Instead, you need to create a bootable disk that can be used to boot a VM or emulated PC. It makes the work easier if there are debugging possibilities. The Qemu [2] and Bochs [3] emulation tools have proven useful for building a virtual environment.
[...]
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
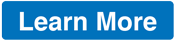
News
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.