Python package simplifies algebraic equations
Programming Snapshot – SymPy
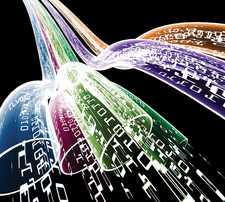
© Lead Image © Andrea Danti, 123RF.com
Whether he's filling the bathtub with water or routing electricity through resistors – Mike Schilli juggles mathematical formulas with the assistance of the Python SymPy package.
I still remember a traumatic event as an elementary school student: A weekend newspaper had set a logic puzzle for kids, the solution of which it promised to publish in the next issue a week later. It involved a bathtub with two taps: one of which filled the tub in 10 minutes, the other in 15. The question was how long would it take to fill the tub if both taps were turned on all the way.
As a little boy, I was absolutely sure that 10 plus 15 equals 25, which is 25 minutes. My father laughed and suggested that couldn't be true, because two taps would fill the bathtub faster than one alone. The next weekend, I was initially triumphant, because there it was – printed in black and white in the following issue – the confirmation that 25 minutes was the correct solution.
But disillusionment followed one week later: After receiving angry reader comments, the newspaper had to admit that it had made a mistake, because it does not take 25 minutes, but only 6 minutes, to fill the tub with both taps. I nearly fell off my chair and decided at that point to become a famous columnist peddling logic puzzles.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
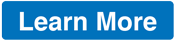
News
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.