Harder than scripting, but easier than programming in C
In 2012, Unix and C veterans Robert Griesemer, Rob Pike, and Ken Thompson released the system-oriented programming language Go under the aegis of Google. For a long time it eked out a niche existence, before eventually becoming the industry standard for system-oriented programming. Today, observers of the Unix scene are rubbing their eyes in disbelief over the number of tools developed in Go.
To name a programming language after an everyday word such as Go seems like a pretty crazy idea from the viewpoint of a search engine operator. After all, search engines actually remove filler words such as "go" from incoming queries. So, when looking for Go programming tips, the recommendation is to search for "Golang" instead, which has also become the accepted name for the language in the community.
Quickly Installed
If you want to try Go, the easiest approach is to grab a package for your favorite distro. On Ubuntu, for example, type:
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
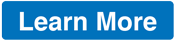
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.