DMX with the Kunbus Revolution Pi Core 3+
Program
The Python script (Listing 3) first loads all the required libraries. The block that follows creates some variables and constants for the offset. As seen in the previous section, values on the RevPi Core can be accessed by their plain text names and by offsets.
Listing 3
dmx_demo.py
01 #!/usr/bin/python3 02 import time 03 import struct 04 05 DEBUG = False 06 07 DMX1_MASTER = 525 08 DMX1_RED = 526 09 DMX1_GREEN = 527 10 DMX1_BLUE = 528 11 12 DMX2_MASTER = 533 13 DMX2_RED = 534 14 DMX2_GREEN = 535 15 DMX2_BLUE = 536 16 17 class RevPi: 18 _rev_pi = open('/dev/piControl0', 'wb+', 0) 19 20 def __init__(self): 21 pass 22 23 class DMX(RevPi): 24 _green = 0 25 _red = 255 26 _blue = 0 27 28 def __init__(self, master, adr_green, adr_red, adr_blue): 29 self.adr_green = adr_green 30 self.adr_red = adr_red 31 self.adr_blue = adr_blue 32 self._rev_pi.seek(master) 33 self._rev_pi.write(struct.pack('<H', 255)) 34 35 def update_color(self, adr, val): 36 self._rev_pi.seek(adr) 37 self._rev_pi.write(struct.pack('<H', val)) 38 39 def set_step(self, mode_val): 40 if mode_val == 1: 41 self._green += 16 42 elif mode_val == 2: 43 self._red += -16 44 elif mode_val == 3: 45 self._blue += 16 46 elif mode_val == 4: 47 self._green += -16 48 elif mode_val == 5: 49 self._red += 16 50 elif mode_val == 6: 51 self._blue += -16 52 53 def do_green(self): 54 if DEBUG: 55 print('GREEN: %d' % self._green) 56 self.update_color(self.adr_green, self._green) 57 58 def do_red(self): 59 if DEBUG: 60 print('RED: %d' % self._red) 61 self.update_color(self.adr_red, self._red) 62 63 def do_blue(self): 64 if DEBUG: 65 print('BLUE: %d' % self._blue) 66 self.update_color(self.adr_blue, self._blue) 67 68 DMX_1 = DMX(DMX1_MASTER, DMX1_GREEN, DMX1_RED, DMX1_BLUE) 69 DMX_2 = DMX(DMX2_MASTER, DMX2_GREEN, DMX2_RED, DMX2_BLUE) 70 71 while 1: 72 for mode in range(1, 7): 73 if DEBUG: 74 print('mode=%d' % mode) 75 for i in range(0, 15): 76 if DEBUG: 77 print('i=%d' % i) 78 DMX_1.set_step(mode) 79 DMX_2.set_step(mode) 80 DMX_1.do_red() 81 DMX_2.do_red() 82 DMX_1.do_green() 83 DMX_2.do_green() 84 DMX_1.do_blue() 85 DMX_2.do_blue() 86 time.sleep(0.05)
With the help of the fcntl
library, the script could be designed in such a way that it determines the offset in the background and uses it instead of the plain text name. The script here avoids this complex procedure by using the offsets directly. Because these values always increase by 1 in the example, you do not have to determine all values by trial and error. A code example demonstrating the use of the plain text names can be found on the Kunbus homepage [5].
Two classes (lines 17 and 23) establish the connection to the RevPi (RevPi
) and control color changes of the lights (DMX
). The open()
command lets you open a connection to the hardware driver and store it in the _rev_Pi
variable. All access to the hardware will then be through this variable.
In the DMX
class, the do_<color>
methods use the update_color()
statement to ensure that the appropriate color is sent to the light. The color is calculated by the set_step()
method and controlled by the mode_val
variable. Because the lamps can only handle fairly coarse dimming, the values for each color are always increased or decreased by 16.
Two lights and three colors allow six possible color transitions, which is why the mode
variable is reset to 1 as soon as it reaches a value of 7 (line 72). The time.sleep(0.05)
command controls the speed of the color transitions. The __init__
method of the DMX
class sets the master dimmer to 100 percent, to make full use of the dimmers for each color.
The while 1
command tells the program to run in an infinite loop, which you can cancel with the Ctrl+C keyboard combination. Inside this loop, the code changes the variable mode
and passes it to the objects for the two lights, turning a particular color on or off. When a color reaches its new value, the mode
variable is incremented by 1. To cycle through a color transition fully, you need to call the set_step()
method 16 times, as in the second for
loop (line 75).
You can launch the demo program with the command
python3 dmx_demo.py
at the RevPi Core's command line. You can find a video on YouTube showing the demo program in action [6].
Conclusions
The DMX module for the Revolution Pi Core 3+ makes creating your own lighting show easy. A little browsing on the Internet reveals many interesting devices that can be controlled by DMX. Besides classic stage lighting, the products also cover lighting systems for home automation. One genuine highlight among the DMX devices is a moving, talking skull [7]. Have fun with your own DMX experiments.
Infos
- DMX lights: https://www.aliexpress.com/item/1005002449259723.html?gatewayAdapt=glo2deu
- RevPi Core 3+: https://revolutionpi.com/revpi-core/
- DMX module user manual: https://www.kunbus.com/files/media/bedienungsanleitungen/DO0262R00-MGW-UM-DMX-EN.pdf
- PuTTY: https://www.putty.org
- Python example: https://revolution.kunbus.com/download/1141/
- Video for the program: https://youtu.be/2i2Httbwc1E
- Talking skull: https://www.hauntedhousecreations.com/full-motion-talking-skull/
« Previous 1 2
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
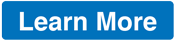
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.