Create a digital spirit level with the ESP32
Spirit Level Program
After testing and performing a trial run with the components of our spirit level without any errors, it's time to take a closer look at the program (Listing 2) for the spirit level. You will find it, along with some other files, in the download section for this article [9]. The first block imports the required libraries (lines 1 to 5). To make the code more readable, the program then defines some constants (lines 6 to 8).
Listing 2
spiritlevel.ino
01 #include "Wire.h" 02 #include <Adafruit_GFX.h> 03 #include <Adafruit_ST7735.h> 04 #include <SPI.h> 05 #include <MPU6050_light.h> 06 #define TFT_CS 17 07 #define TFT_RST 14 08 #define TFT_DC 2 09 10 Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST); 11 MPU6050 mpu(Wire); 12 13 void setup(void) { 14 Wire.begin(); 15 mpu.begin(); 16 mpu.calcOffsets(); 17 tft.initR(INITR_BLACKTAB); 18 tft.fillScreen(ST77XX_BLACK); 19 } 20 21 int x, y, xold, yold; 22 23 void loop() { 24 mpu.update(); 25 x=floor(mpu.getAngleX()); 26 y=floor(mpu.getAngleY()); 27 if ((x!=xold) or (y!=yold)) { 28 tft.setTextSize(1, 3); 29 tft.setCursor(20, 0); 30 tft.setTextColor(ST77XX_BLUE); 31 tft.print("2D-Wasserwaage"); 32 tft.fillCircle(64-x, 80+y, 12, ST77XX_BLACK); 33 tft.fillCircle(64-x, 80+y, 5, ST77XX_YELLOW); 34 tft.drawCircle(64, 80, 7, ST77XX_RED); 35 tft.drawCircle(64, 80, 27, ST77XX_RED); 36 tft.drawCircle(64, 80, 47, ST77XX_RED); 37 tft.drawLine(64, 30, 64, 130, ST77XX_RED); 38 tft.drawLine(14, 80, 114, 80, ST77XX_RED); 39 tft.fillRect(0, 142, 160, 30, ST77XX_BLACK); 40 tft.setTextSize(2); 41 tft.setTextColor(ST77XX_GREEN); 42 tft.setCursor(0, 142); 43 tft.print("x="); 44 tft.println(x); 45 tft.setCursor(64, 142); 46 tft.print("y="); 47 tft.println(y); 48 } 49 xold=x; 50 yold=y; 51 delay(50); 52 }
Lines 10 and 11 define the objects that we will use to address the display (tft
) and the sensor (mpu
). The setup()
function starting in line 13 initializes all of the objects, with the calcOffsets()
method to calibrate the sensor. After starting the program, it will take a while for the values to settle. The variable definitions for the measured value of the X and Y axis then follow (line 21). The xold
and yold
variables are used to determine whether the values changed since the last measurement. If so, the program updates the contents of the display. This procedure reduces the volume of data sent to the display to the required minimum.
The loop()
function calls the mpu.update()
method to start a measurement (line 24). Then the mpu.getAngleX()
and mpu.getAngleY()
methods read the measurement values. The library returns floating-point values, which the floor()
function converts to integers. Some sensor accuracy is lost in the process, but as a positive side effect, the values will not fluctuate as much. At this point, there are certainly many solutions that offer more accurate results. But for our spirit level, this simple procedure is perfectly OK. Just bear in mind that with more overhead you can tickle more accurate values out of the sensor.
The commands in the if
statement starting in line 27 update the display. What is important to note here is that the program always drops new display information on top of the existing information. This means that you can't read older display information after some time because everything is filled up. Erasing the entire display before each write isn't a good idea either because it causes annoying flickering.
The solution is to selectively edit the areas that change. The command
<C>tft.fillRect(0,142,160,30,ST77XX_<C><C> BLACK)<C>
in line 39 erases just the part where the measured values are shown. Parts that remain constant, such as the heading, are overwritten by the program. Apart from this, the code in this section is pretty much self-explanatory. If in doubt, consult the library documentation [5].
At the end of the loop()
function starting in line 40, the program copies the values of the x
and y
variables to xold
and yold
. This is followed by a delay()
to the flow before the program starts the next round of the loop. A YouTube video [10] shows you how the program works.
Conclusions
This article shows how to build a digital spirit level with simple materials. All in all, there are still many options for increasing the sensor's accuracy. A 3D printed housing for the technology would massively improve the stability of the setup.
The sample program, which is fairly simple, makes it easy to implement quite a bit more accuracy and functionality. The combination of ESP32 and the display allows for many more interesting projects to be built. All told, this is a neat project that you can learn a great deal from. I hope you makers out there enjoy building your personal spirit level.
Infos
- MPU6050 module: https://www.az-delivery.de/en/products/gy-521-6-achsen-gyroskop-und-beschleunigungssensor
- Data sheet for MPU6050: https://invensense.tdk.com/wp-content/uploads/2015/02/MPU-6000-Datasheet1.pdf
- NodeMCU ESP32 development kit: https://www.az-delivery.de/en/products/esp-32-dev-kit-c-v4
- 1.8 inch TFT color display: https://www.az-delivery.de/en/products/1-8-zoll-spi-tft-display
- Adafruit GFX library: https://learn.adafruit.com/adafruit-gfx-graphics-library
- Adafruit GFX library (Quellen): https://github.com/adafruit/Adafruit-GFX-Library
- TFT LCD library: https://www.arduino.cc/en/Reference/TFTLibrary
- Adafruit ST7735 library: https://github.com/adafruit/Adafruit-ST7735-Library
- Source code for this article: ftp://ftp.linux-magazine.com/pub/listings/MakerSpace/
- Spirit level in action [in German]: https://youtu.be/ib4KpretMa8:
« Previous 1 2
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
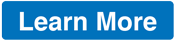
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.