Explore the possibilities of Ethereum's smart contract feature
Digital currencies have had quite a lot of buzz around them in recent years, with Bitcoin being the most famous. Satoshi Nakamoto, the mysterious creator of Bitcoin, had the idea to use Bitcoin as a store of value, like gold. For that reason, Bitcoin was designed for the limited purpose of trading and holding value.
When Vitalik Buterin and others designed the first version of Ethereum [1], they wanted to extend the blockchain concept to include other kinds of transactions. Ethereum is built around the concept of a smart contract. A smart contract is a program embedded in the blockchain that automatically manages, controls, and documents actions taken for a predetermined purpose. A smart contract allows the creator to implement a contract or agreement without the need for traditional oversight and enforcement.
Bitcoin is thought to have some rudimentary support for smart contracts in the context of its role as a digital currency – to support features such as escrows and multisignature accounts, but Ethereum expands the concept to support a much wider range of transactions and arrangement. For instance, Ethereum was the first blockchain technology to support non-fungible tokens (NFTs) [2], and Ethereum is still the most popular tool for creating NFTs.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
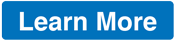
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.