Overview of the Serial Communication Protocol
The word "serial" in terms of computers might bring several things to mind. In modern times, it might be the universal serial bus (USB). Not too long ago, it might have brought to mind a 9-pin connector on the back of your desktop – or the even bigger 25-pin connector a bit before that. Different, still, the term might bring up memories of modems, printers, or peripherals connected to specialty computers. If you look at early mainframes, the serial port was the main interface to a text terminal and thus the human interface to the computer.
Some of these usages have been superseded by newer or different technologies, but serial is still alive and well. Although not as common on today's computer equipment, serial communication is far from gone, and its availability can provide some interesting possibilities for talking to unique hardware.
Basic Principles
In the simplest sense, an electric circuit used for communication just uses a completed circuit to represent a 1 and a broken circuit to represent a 0. See the "Telegraphy" sidebar for how this worked in real life for years. The limitation of a simple telegraphy circuit is that for each signal you want to send, you need a dedicated wire.
[...]
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
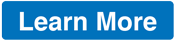
News
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.