A good cents exit survey
To generate useful feedback for live programs at the Fort Worth Museum of Science & History's Noble Planetarium on the campus of Central Texas College in Killeen, Texas, we conceived, designed, and built a custom feedback system centered around pennies. The goals were (1) ease of use for both guests and staff, (2) a fast participation process, and (3) immediate feedback to the presenters and managers.
The Guest Experience
When you attend a planetarium show at the Noble Planetarium, you are handed a penny on the way into the theater. As a part of the show, we ask you to hold out the penny at arm's length and imagine that you are looking through Abraham Lincoln's eye. That approximates the field of view of the Hubble Space Telescope, which is the frame of reference for the remainder of the program.
As you exit the theater, the presenter has rolled out an interesting device that looks sort of like a coin bank (Figure 1). You are invited to return the penny in one of five slots to indicate how much you liked the show. As the pennies are returned, they are tallied by the machine; once everyone has exited, the presenter submits the counts, which are immediately visible on an internal web page.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
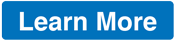
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.