Solving a classic interview problem with Go
The TechLead [1], Patrick Shyu, is a YouTube celebrity whose videos I like to watch. The former Google employee, who has also completed a gig at Facebook, talks about his experiences as a software engineer in Silicon Valley in numerous episodes on his channel (Figure 1). His trademark is to hold a cup of coffee in his hand and sip it pleasurably every now and then while he repeatedly emphasizes that he's the "tech lead." That's how Google refers to lead engineers who set the direction for the other engineers on the team. The first-line managers there traditionally stay out of technical matters and focus primarily on staffing and motivating their reports.
One episode on the TechLead channel is about typical questions asked at interviews at Google, of which the former employee says he has conducted hundreds. In this Snapshot issue, we'll tackle one of the quiz questions that he allegedly invented himself and kept asking, a slightly modified version of the flood fill problem [2]. The latter is so well-known that by now any candidate can rattle off the solution blindfolded. That's why Google has removed it from the list of questions, and the TechLead created his own version [3].
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
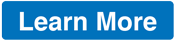
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.