Overview of the Serial Communication Protocol
The word "serial" in terms of computers might bring several things to mind. In modern times, it might be the universal serial bus (USB). Not too long ago, it might have brought to mind a 9-pin connector on the back of your desktop – or the even bigger 25-pin connector a bit before that. Different, still, the term might bring up memories of modems, printers, or peripherals connected to specialty computers. If you look at early mainframes, the serial port was the main interface to a text terminal and thus the human interface to the computer.
Some of these usages have been superseded by newer or different technologies, but serial is still alive and well. Although not as common on today's computer equipment, serial communication is far from gone, and its availability can provide some interesting possibilities for talking to unique hardware.
Basic Principles
In the simplest sense, an electric circuit used for communication just uses a completed circuit to represent a 1 and a broken circuit to represent a 0. See the "Telegraphy" sidebar for how this worked in real life for years. The limitation of a simple telegraphy circuit is that for each signal you want to send, you need a dedicated wire.
[...]
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
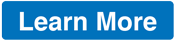
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.