Monitor hard disk usage with Go
Signed vs. Unsigned
The Statfs()
function returns the remaining disk space in line 64 as uint64
, (i.e., as an unsigned 64-bit integer that can never assume a negative value). However, the difference between two of these values can definitely be negative.
If you simply subtract both values from each other, you might be surprised to see that Go (like other languages) returns absolutely insane values for the difference if the subtrahend (the number we're subtracting) turns out to be larger than the minuend (the number we're subtracting from). The result should be negative in this case, but instead you get very large positive values. Without any help, Go assumes that the result of an operation with two unsigned integers is also an unsigned integer. The solution: Typecasting with int(x-y)
makes it clear to Go that the result of the difference of two uint64
values x
and y
is in fact a signed value.
But before line 51 divides the difference by an empirically determined value of 100,000, resulting in a floating point value between
and 1
for the average disk performance, line 48 first needs to convert the result type to float64
. Now, if the velocity is greater than 1
or less than -1
, the if-else construct from line 53 squashes it into the range between -1
and +1
.
All that remains for the main program in Listing 1 to do is to determine the current directory (line 10), start the user interface (UI) (line 17), and close it in any situation with the defer
statement in line 18 as soon as the main program stops running.
On the Screen
Listing 2 shows how the program conjures up the UI with the speedometer display in a terminal window using the tried and tested termui project from GitHub. It defines three stacked widgets: a paragraph widget to display the program name Disk Speedo v1.0
, a progress bar of the Gauge
type, and a pie chart of the PieChart
type that forms the speedometer.
Listing 2
ui.go
01 package main 02 import ( 03 "math" 04 tui "github.com/gizak/termui/v3" 05 "github.com/gizak/termui/v3/widgets" 06 ) 07 type UI struct { 08 Gauge *widgets.Gauge 09 Pie *widgets.PieChart 10 Head *widgets.Paragraph 11 } 12 13 func NewUI() *UI { 14 h := widgets.NewParagraph() 15 h.TextStyle.Fg = tui.ColorBlack 16 h.SetRect(6, 1, 30, 2) 17 h.Text = "Disk Speedo v1.0" 18 h.Border = false 19 g := widgets.NewGauge() 20 g.SetRect(2, 2, 28, 5) 21 g.Percent = 0 22 g.BarColor = tui.ColorRed 23 p := widgets.NewPieChart() 24 p.SetRect(-10, 5, 40, 20) 25 p.Border = false 26 p.Data = fract(0) 27 p.AngleOffset = -1.5 * math.Pi 28 return &UI{Gauge: g, 29 Pie: p, Head: h} 30 } 31 32 func (ui UI) Run() chan bool { 33 done := make(chan bool) 34 err := tui.Init() 35 if err != nil { 36 panic("termui init failed") 37 } 38 39 go func() { 40 events := tui.PollEvents() 41 for { 42 select { 43 case e := <-events: 44 switch e.ID { 45 case "q", "<C-c>": 46 done <- true 47 return 48 } 49 } 50 } 51 }() 52 return done 53 } 54 55 func (ui UI) Close() { 56 tui.Close() 57 } 58 59 func (ui UI) Update( 60 level int, 61 speed float64) { 62 ui.Gauge.Percent = level 63 ui.Pie.Colors = []tui.Color{ 64 tui.ColorBlack, tui.ColorRed} 65 if speed < 0 { 66 ui.Pie.Colors[1] = 67 tui.ColorGreen 68 speed = -speed 69 } 70 ui.Pie.Data = fract(speed) 71 tui.Render(ui.Head, ui.Gauge, 72 ui.Pie) 73 } 74 75 func fract( 76 val float64) []float64 { 77 num := (1 - val) * 100 78 denom := val * 100 79 return []float64{num, denom} 80 }
The NewUI()
constructor from line 13 defines the widgets, including their dimensions, geometric positions, and colored bits, and returns an initialized structure of the UI
type (defined in line 7). The main program uses this later to call method-style functions such as Run()
(from line 32) and to give them the context of the previously initialized widgets – object orientation Go-style.
Listening on the Channels
The Run()
function from line 32 onwards starts the termui interface in a parallel Goroutine from line 39 onwards. Like every UI, it also continuously delivers events such as keystrokes or mouse clicks that need to be intercepted and processed in order to give the illusion of a seamless user interaction.
The infinite loop from line 41 waits with its select
statement for the user to press either Q or Ctrl+C. In this case, it sends the value true
into a newly created channel, done
, to the listening main program, causing it to stop all operations. To do this, the main program simultaneously monitors – in the select statement from line 32 of Listing 1 – whether the UI has reported the end of the program or, alternatively, the ticking seconds timer (Listing 1, line 35) has expired. If the timer has expired, the program moves on to the next round, fetches new values for the disk fill level, and displays them.
In general terms, Listing 2 abstracts the features of the termui library and encapsulates them from the main flow. When it's time to pack up shop, the main program simply calls the Close()
function (Listing 2 from line 55) to wrap up the terminal UI, which in turn triggers a Close()
call in the termui library.
« Previous 1 2 3 Next »
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
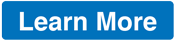
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.