Fast and reliable programs with OCaml
Pattern Matching
OCaml's version of switch
is match
. Here, low numbers print as words, but anything else uses the built-in string_of_int
function to display a decimal:
let to_string x = match x with | 1 -> "one" | 2 -> "two" | n -> string_of_int n
You can also match on strings, variants, records, and other complex values. OCaml will always check that you have covered every case. This is the key to its reliability.
Pattern matching can also be used in anonymous functions, like this:
let to_string = function | 1 -> "one" | 2 -> "two" | n -> string_of_int n
Anonymous functions are like lambda expressions in Python; you'll often see this shorter form.
Data Structures
OCaml allows you to define both variants (unions representing a choice of alternatives) and records (structs or named tuples holding several values at once). For example, the result of a download can be declared as a variant:
type download_result = | Success of filepath | Network_error of string | Aborted_by_user
This ensures that everywhere a URL downloads, it also handles a failed download (typically by displaying the error to the user) or a canceled download (not an error).
It's used as follows:
match download url with | Success file -> process file | Network_error msg -> alert msg | Aborted_by_user -> ()
Notice that each case can only access the appropriate value: You can't accidentally try to use file
if the download failed or display msg
if it succeeded.
A record joins together several pieces of information:
type program = { name : string; homepage : url option; }
Records and variants can be combined. In fact, the type url option
in this record is itself a variant, because the homepage
field can be either None
or Some
URL. By making optional types explicit, OCaml completely avoids Java's dreaded NullPointerException
(or C's segfault
).
OCaml also supports polymorphic (generic) types:
type 'a result = | Success of 'a | Error of exn
These allow you to construct whole families of types (e.g., int
result, string
result, or program
result), while still writing generic code that can work on any of them, such as this function to return a result's value (or throw its exception):
let success = function | Success value -> value | Error ex -> raise ex
You don't have to declare variants before use; you can use the syntax `<tag>
to let OCaml infer variant types automatically (examples below).
Loops
An unusual feature of functional programming is that loops are often written in a tail-recursive style (Listing 1). This prompts the user with a question, returning `yes
if the user enters y or yes and so on.
Listing 1
Tail-Recursive Loops
If the user doesn't provide a valid response, it calls itself recursively to ask again. Because this recursive call is always the last thing the function does, OCaml does not need to store the return address on the stack, and you can loop any number of times without running out of memory. Effectively, the function call turns into a goto.
OCaml does support more traditional for
and while
loops, too, but the recursive form can be more elegant.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
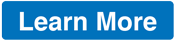
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.